【Unity】円形UIのクリックイベントを実現する方法
Unityで円形のUI Imageをクリックしたときにイベントを実行するためには、以下の手順で設定できます
手順のまとめ
1. テクスチャ設定
- Unityエディタで使用する円形の画像(アルファチャンネル付きPNG)を選択します。
- インスペクターウィンドウで、以下の設定を行います。
- Texture Type: Sprite(2D and UI)
- AdvancedのRead/Write Enabled: チェックを入れる
- Alpha Is Transparency: 必要に応じてチェックを入れる
- 設定を変更したら、Applyボタンをクリックして適用します。
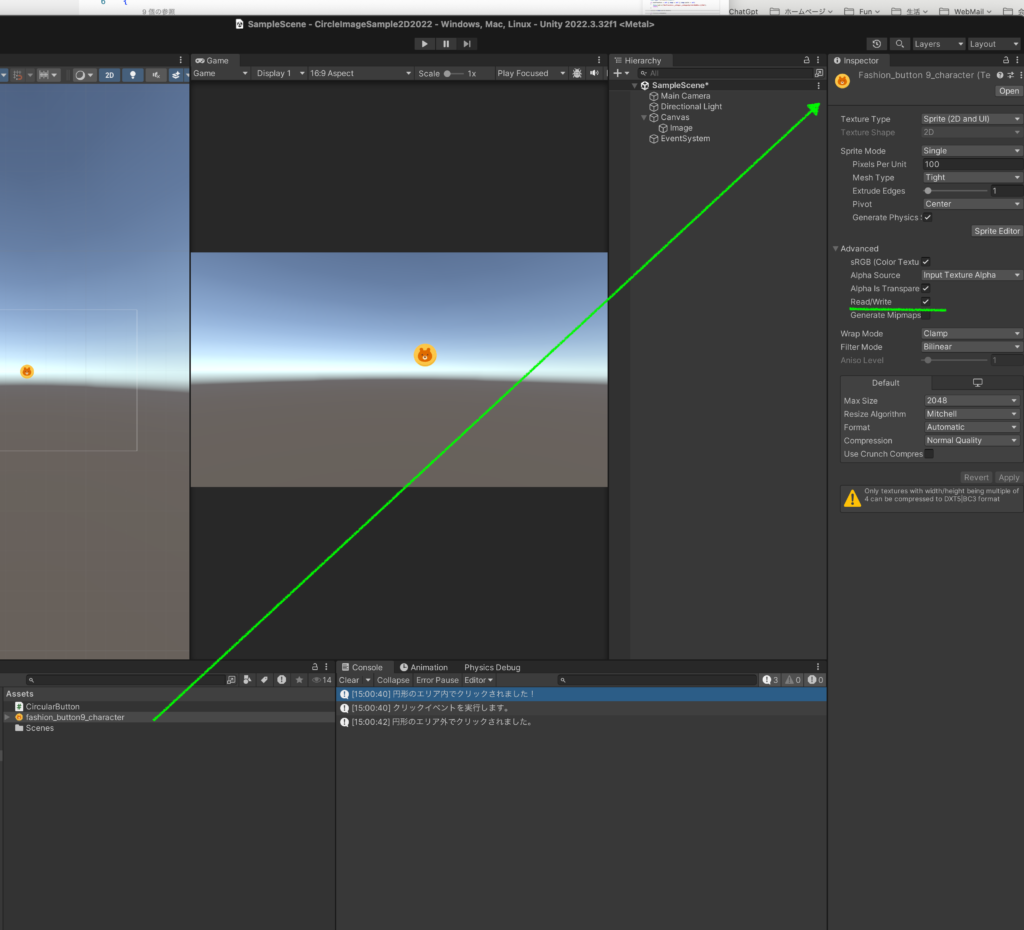
2. スクリプトの作成
以下のスクリプトを作成し、円形のクリック判定を行います。
using UnityEngine;
using UnityEngine.EventSystems;
using UnityEngine.UI;
public class CircularButton : MonoBehaviour, IPointerClickHandler
{
private RectTransform rectTransform;
private Texture2D texture;
private Color[] pixels;
void Start()
{
rectTransform = GetComponent<RectTransform>();
Image image = GetComponent<Image>();
if (rectTransform == null || image == null || image.sprite == null)
{
Debug.LogError("RectTransformまたはImageまたはImageのSpriteが見つかりません。");
return;
}
texture = image.sprite.texture;
if (!texture.isReadable)
{
Debug.LogError("テクスチャが読み取り可能ではありません。テクスチャのインポート設定で「Read/Write Enabled」を有効にしてください。");
return;
}
pixels = texture.GetPixels();
}
public void OnPointerClick(PointerEventData eventData)
{
Vector2 localMousePosition;
RectTransformUtility.ScreenPointToLocalPointInRectangle(rectTransform, eventData.position, eventData.pressEventCamera, out localMousePosition);
Vector2 pivotOffset = new Vector2(rectTransform.rect.width * rectTransform.pivot.x, rectTransform.rect.height * rectTransform.pivot.y);
Vector2 textureCoord = localMousePosition + pivotOffset;
float widthScale = texture.width / rectTransform.rect.width;
float heightScale = texture.height / rectTransform.rect.height;
int x = Mathf.Clamp((int)(textureCoord.x * widthScale), 0, texture.width - 1);
int y = Mathf.Clamp((int)(textureCoord.y * heightScale), 0, texture.height - 1);
Color pixelColor = pixels[y * texture.width + x];
if (pixelColor.a > 0.1f) // アルファ値が0.1以上のピクセルをクリック可能とする
{
Debug.Log("円形のエリア内でクリックされました!");
ExecuteYourEvent();
}
else
{
Debug.Log("円形のエリア外でクリックされました。");
}
}
private void ExecuteYourEvent()
{
// ここにクリック時に実行したい処理を記述
Debug.Log("クリックイベントを実行します。");
}
}
3. UIの設定
- Canvasの設定
Canvas
を作成し、Render Mode
をScreen Space - Overlay
に設定します。
- Imageの設定
Canvas
内にImage
オブジェクトを作成します。Image
のSource Image
に円形の画像を設定します。
- スクリプトのアタッチ
- 上記の
CircularButton
スクリプトをImage
オブジェクトにアタッチします。
- 上記の
スクリプトの動作
- スクリプトの
Start
メソッドで、Image
オブジェクトのRectTransform
とImage
コンポーネント、およびそのテクスチャデータを取得します。 OnPointerClick
メソッドで、クリック位置をローカル座標に変換し、画像のピクセルデータを使用してクリック判定を行います。- アルファ値が0.1以上のピクセルがクリックされた場合のみ、イベントを実行します。
動作確認
- プレイモードに入り、円形のエリア内でクリックすると、指定したイベントが実行されることを確認します。
- 円形のエリア外でクリックすると、クリックイベントは実行されません。
この手順で、円形のエリア内でのみクリックを有効にし、イベントを実行する方法が完成します。
ディスカッション
コメント一覧
まだ、コメントがありません