WindowsFormsアプリでのMVCパターンサンプル
以下は、WindowsFormsアプリでMVCパターンを実装するための簡単なサンプルです
目次
MVCとは
MVCは、アプリケーションの機能を3つの役割に分割することで、アプリケーションの保守性や拡張性を向上するデザインパターンです。Modelは、アプリケーションのデータを表し、Viewは、ユーザーインターフェイスを表します。Controllerは、ユーザーの入力を処理し、ModelとViewを連携してアプリケーションの機能を実現します。
クラス図
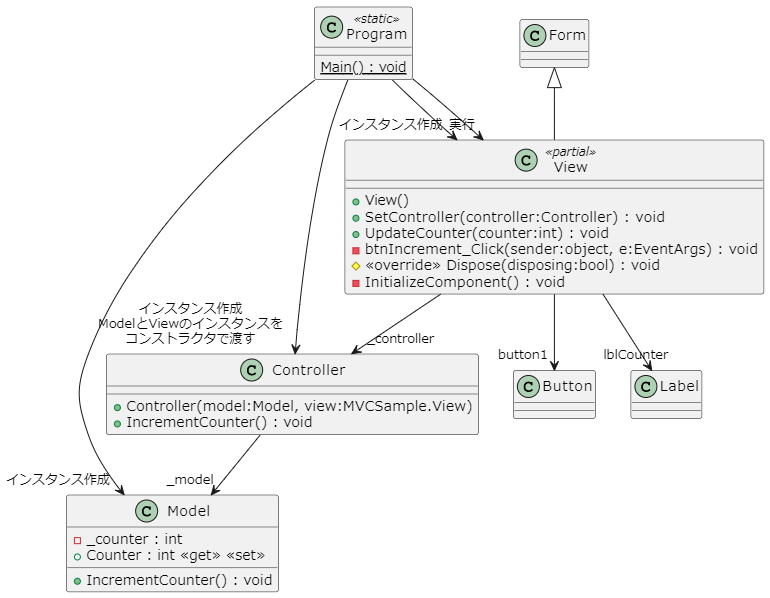
サンプル概要
このサンプルでは、Viewにはカウンターの値を表示するラベルと、カウンターをインクリメントするボタンがあります。ボタンがクリックされると、ControllerがModelを更新し、Viewを更新します。Viewは、Controllerにアクセスするために、SetControllerメソッドを使用しています。このメソッドにより、ControllerはViewにバインドされます。
実行結果
フォームデザイン
ラベルとボタンを1つずつ配置します
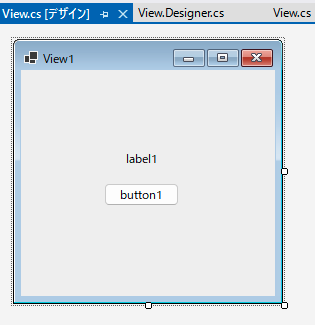
ボタンのクリックのイベントを登録します
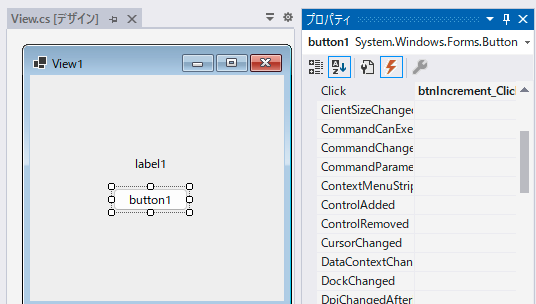
ソリューション
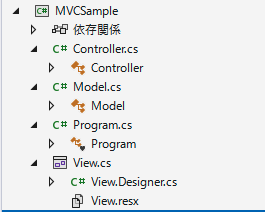
コード
Model
namespace MVCSample
{
public class Model
{
private int _counter;
public int Counter
{
get { return _counter; }
set { _counter = value; }
}
public void IncrementCounter()
{
_counter++;
}
}
}
View
namespace MVCSample
{
public partial class View : Form
{
private Controller _controller;
public View()
{
InitializeComponent();
}
public void SetController(Controller controller)
{
_controller = controller;
}
public void UpdateCounter(int counter)
{
lblCounter.Text = counter.ToString();
}
private void btnIncrement_Click(object sender, EventArgs e)
{
_controller.IncrementCounter();
}
}
}
namespace MVCSample
{
partial class View
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.button1 = new System.Windows.Forms.Button();
this.lblCounter = new System.Windows.Forms.Label();
this.SuspendLayout();
//
// button1
//
this.button1.Location = new System.Drawing.Point(83, 113);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(75, 23);
this.button1.TabIndex = 3;
this.button1.Text = "button1";
this.button1.UseVisualStyleBackColor = true;
this.button1.Click += new System.EventHandler(this.btnIncrement_Click);
//
// lblCounter
//
this.lblCounter.AutoSize = true;
this.lblCounter.Location = new System.Drawing.Point(102, 81);
this.lblCounter.Name = "lblCounter";
this.lblCounter.Size = new System.Drawing.Size(38, 15);
this.lblCounter.TabIndex = 2;
this.lblCounter.Text = "label1";
//
// View
//
this.AutoScaleDimensions = new System.Drawing.SizeF(7F, 15F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(254, 226);
this.Controls.Add(this.button1);
this.Controls.Add(this.lblCounter);
this.Name = "View";
this.Text = "View1";
this.Click += new System.EventHandler(this.btnIncrement_Click);
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private Button button1;
private Label lblCounter;
}
}
Controller
namespace MVCSample
{
public class Controller
{
private Model _model;
private View _view;
public Controller(Model model, View view)
{
_model = model;
_view = view;
_view.SetController(this);
}
public void IncrementCounter()
{
_model.IncrementCounter();
_view.UpdateCounter(_model.Counter);
}
}
}
Program.cs
namespace MVCSample
{
internal static class Program
{
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
// To customize application configuration such as set high DPI settings or default font,
// see https://aka.ms/applicationconfiguration.
ApplicationConfiguration.Initialize();
Model model = new();
View view = new();
Controller controller = new(model, view);
Application.Run(view);
}
}
}
ディスカッション
コメント一覧
_controller.IncrementCounter();にて、以下のようなエラーが出ます。解決策はありますか?
System.NullReferenceException: ‘オブジェクト参照がオブジェクト インスタンスに設定されていません。’
当方では動作しました。
詳細が無いため推測ですがProgram.csの編集をお忘れなのではないでしょうか。