【C#】WindowsFormsアプリでのFormクラスとControlsプロパティの関係
FormクラスのControlsプロパティは、UIのコントロール(ボタンやラベルなど)を格納するリストと考えられます
シミュレートしたコードを元に仕組みについて考えてみましょう
目次
基本サンプル
実行結果(ダミー)
Formクラスのインスタンスは、1つのコントロール(ボタン)を所持(持っている)しています
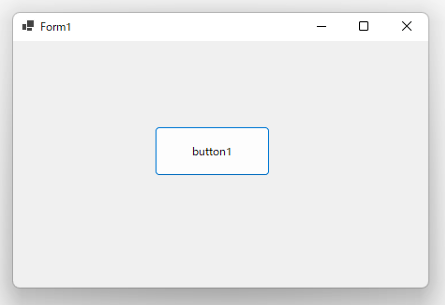
シミュレートしたコード
Form1 form1 = new Form1();
class Form1 : Form
{
public Form1()
{
Button button = new Button();
Controls.Add(button);
}
}
class Form
{
// Controlsに登録されたコントロールは、フォーム上に表示される
public List<Control> Controls { get; set; } = new();
}
class Button : Control
{
}
class Control
{
}
このコードは、簡単なWindowsフォームアプリケーションをシミュレートするためのC#のコードの一部です。以下では、コードの主要な部分とそれぞれのクラスを説明します。
Form1 form1 = new Form1();
: これはForm1
クラスのインスタンスを作成し、form1
という名前の変数に割り当てています。このインスタンスは、後で説明するように、Windowsフォームの基本となる要素を含むフォームを表します。Form1
クラス:Form1
クラスは、Form
クラスを継承しています。これはWindowsフォームの親ウィンドウとして機能し、ユーザーインターフェース(UI)コントロールを含むことができます。Form1
クラスのコンストラクタでは、Button
コントロールを作成し、このフォームに追加しています。Button
は後で説明します。Form
クラス:Form
クラスは、UIコントロールを管理するための基本的なクラスです。このクラスには、UIコントロールのリストを格納するControls
プロパティが含まれています。Controls
プロパティは、このフォームに追加されたすべてのUIコントロールを保持します。Button
クラス:Button
クラスは、ボタンコントロールを表すクラスです。ボタンはユーザーがクリックできる要素であり、通常は何らかのアクションをトリガーします。このクラスはControl
クラスを継承しています。Control
クラス:Control
クラスは、UIコントロールの基本クラスで、すべてのUIコントロールが共通で持つプロパティやメソッドを提供します。Button
クラスと同様に、他のUIコントロールもこのクラスを継承して機能を拡張します。
このコードは、Windowsフォームアプリケーションの基本的な構造をシンプルに模倣しており、Form1
フォームには少なくとも1つのボタンが追加されています。これらのクラスは、実際のWindowsフォームアプリケーションの基本要素であるフォームとUIコントロールを理解するための基本的な概念を示しています。実際のアプリケーションでは、さらに多くの機能やイベント処理が含まれることが一般的です。
クラス図
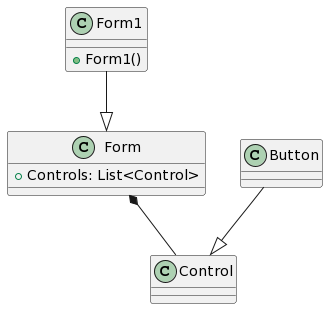
@startuml
class Form1 {
+Form1()
}
class Form {
+Controls: List<Control>
}
class Button {
}
class Control {
}
Form1 --|> Form
Form *-- Control
Button --|> Control
@enduml
実際のコードに近いサンプル
using System;
using System.Windows.Forms;
namespace MyWindowsApp
{
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
// フォームにボタンコントロールを追加
Button myButton = new Button();
myButton.Text = "クリックしてください";
myButton.Click += MyButton_Click; // ボタンのクリックイベントハンドラを設定
Controls.Add(myButton); // フォームにボタンを追加
}
private void MyButton_Click(object sender, EventArgs e)
{
MessageBox.Show("ボタンがクリックされました!");
}
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new MainForm());
}
}
}
ContolクラスにNameプロパティを追加してみる
コード
Form1 form1 = new Form1();
class Form1 : Form
{
public Form1()
{
Button button = new Button();
button.Name = "ボタン1";
Controls.Add(button);
foreach (var control in Controls)
{
Console.WriteLine(control.Name);
}
}
}
class Form
{
// Controlsに登録されたコントロールは、フォーム上に表示される
public List<Control> Controls { get; set; } = new();
}
class Button : Control
{
}
class Control
{
public string Name { get; set; }
}
実行結果
ボタン1
Labelクラスを追加してみる
コード
Form1 form1 = new Form1();
class Form1 : Form
{
public Form1()
{
Button button = new Button();
button.Name = "ボタン1";
Controls.Add(button);
Label label = new Label();
label.Name = "ラベル1";
Controls.Add(label);
foreach (var control in Controls)
{
Console.WriteLine(control.Name);
}
}
}
class Form
{
// Controlsに登録されたコントロールは、フォーム上に表示される
public List<Control> Controls { get; set; } = new();
}
class Button : Control
{
}
class Label : Control
{
}
class Control
{
public string Name { get; set; }
}
実行結果
ボタン1
ラベル1
クラス図
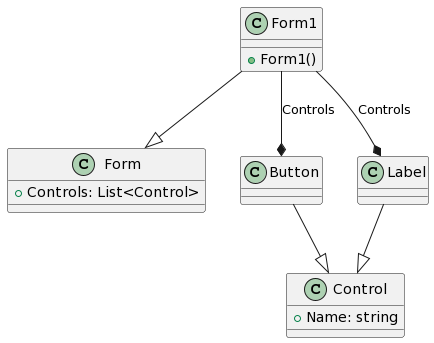
@startuml
class Form1 {
+Form1()
}
class Form {
+Controls: List<Control>
}
class Button {
}
class Label {
}
class Control {
+ Name: string
}
Form1 --|> Form
Form *-- Control
Button --|> Control
Label --|> Control
@enduml
ディスカッション
コメント一覧
まだ、コメントがありません