Newtonsoft.JsonからSystem.Text.Jsonへの移行:C#での天気情報アプリケーション開発
概要
この資料では、C#を使用してWindowsフォームアプリケーションで天気情報を表示する方法を学びます。特に、JSONデータの解析において、Newtonsoft.JsonからSystem.Text.Jsonへの移行に焦点を当てています。初心者にも分かりやすいように、詳細なコメントとともにコードを解説します。
1. はじめに
C#でJSONデータを解析するために使用されるライブラリとして、Newtonsoft.Jsonが広く知られていますが、.NET Core 3.0以降、System.Text.Jsonが標準ライブラリとして提供されています。この資料では、天気情報を取得し表示するアプリケーションを作成しながら、Newtonsoft.JsonからSystem.Text.Jsonへの移行方法を学びます。
2. 前提条件
- Visual Studioがインストールされていること
- 基本的なC#の知識
- Windowsフォームアプリケーションの基本的な理解
3. プロジェクトの作成
- Visual Studioを開き、新しいWindowsフォームアプリケーションプロジェクトを作成します。
Windowsフォームアプリ(.NET版)を選択します
プロジェクト名はWeatherCheckerByDotNetにします - プロジェクトに必要なコントロール(コンボボックス、ピクチャーボックスなど)を追加します。
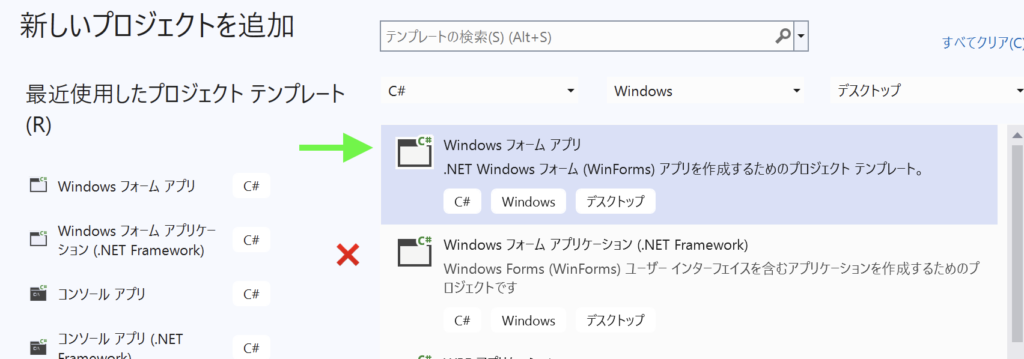
4. Newtonsoft.Jsonを使用したサンプルコード
まず、Newtonsoft.Jsonを使用したコードを見てみましょう。
using System;
using System.Collections.Generic;
using System.Net.Http;
using System.Windows.Forms;
using Newtonsoft.Json.Linq;
namespace WeatherChecker
{
public partial class Form1 : Form
{
Dictionary<string, string> cityNames;
public Form1()
{
InitializeComponent();
this.cityNames = new Dictionary<string, string>
{
{ "東京都", "3" },
{ "大阪府", "1" },
{ "愛知県", "2" },
{ "福岡県", "10" }
};
foreach (KeyValuePair<string, string> data in this.cityNames)
{
areaBox.Items.Add(data.Key);
}
}
private async void CitySelected(object sender, EventArgs e)
{
string cityCode = cityNames[areaBox.Text];
string url = $"天気予報URLcity={cityCode}";
HttpClient client = new HttpClient();
string result = await client.GetStringAsync(url);
JObject jobj = JObject.Parse(result);
string todayWeatherIcon = (string)jobj["url"];
weatherIcon.ImageLocation = todayWeatherIcon;
}
private void ExitMenuClicked(object sender, EventArgs e)
{
this.Close();
}
}
}
5. System.Text.Jsonを使用したリファクタリング
次に、Newtonsoft.JsonをSystem.Text.Jsonに置き換えたコードを示します。
using System;
using System.Collections.Generic;
using System.Net.Http;
using System.Text.Json;
using System.Text.Json.Serialization;
using System.Windows.Forms;
namespace WeatherCheckerByDotNet
{
public partial class Form1 : Form
{
Dictionary<string, string> cityNames;
public Form1()
{
InitializeComponent();
this.cityNames = new Dictionary<string, string>
{
{ "東京都", "3" },
{ "大阪府", "1" },
{ "愛知県", "2" },
{ "福岡県", "10" }
};
foreach (KeyValuePair<string, string> data in this.cityNames)
{
areaBox.Items.Add(data.Key);
}
}
// 都市が選択されたときに呼び出されるメソッド
private async void CitySelected(object sender, EventArgs e)
{
string cityCode = cityNames[areaBox.Text];
string url = $"https://and-idea.sbcr.jp/sp/90261/weatherCheck.php?city={cityCode}";
HttpClient client = new HttpClient();
string result = await client.GetStringAsync(url);
// JSONデータをWeatherInfoクラスにデシリアライズ
WeatherInfo weatherInfo = JsonSerializer.Deserialize<WeatherInfo>(result);
// 天気情報からアイコンのURLを取り出して表示
string todayWeatherIcon = weatherInfo.Url;
weatherIcon.ImageLocation = todayWeatherIcon;
}
private void ExitMenuClicked(object sender, EventArgs e)
{
this.Close();
}
}
// 天気情報を表すクラス
public class WeatherInfo
{
[JsonPropertyName("city")]
public string City { get; set; }
[JsonPropertyName("weather")]
public string Weather { get; set; }
[JsonPropertyName("location")]
public Location Location { get; set; }
[JsonPropertyName("percent")]
public string Percent { get; set; }
[JsonPropertyName("humidity")]
public string Humidity { get; set; }
[JsonPropertyName("temp")]
public string Temp { get; set; }
[JsonPropertyName("wind")]
public string Wind { get; set; }
[JsonPropertyName("pressure")]
public string Pressure { get; set; }
[JsonPropertyName("url")]
public string Url { get; set; }
}
// 位置情報を表すクラス
public class Location
{
[JsonPropertyName("longitude")]
public string Longitude { get; set; }
[JsonPropertyName("latitude")]
public string Latitude { get; set; }
}
}
主な変更点
- System.Text.Jsonを使用:
JObject
の代わりにJsonDocument
とJsonElement
を使用し、JsonSerializer
を使ってデシリアライズしています。 - デシリアライズのためのクラス作成:
WeatherInfo
とLocation
クラスを作成し、JSONデータをこれらのクラスにマッピングします。
6. 結論
System.Text.Jsonは、Newtonsoft.Jsonに比べて軽量で高速です。標準ライブラリであるため、追加のパッケージをインストールする必要がなく、.NET Core 3.0以降のプロジェクトで推奨されます。この資料を通じて、JSONデータの解析方法や、ライブラリの移行手順を学ぶことができました。
参考文献
この資料を使って、Newtonsoft.JsonからSystem.Text.Jsonへの移行に自信を持ち、C#でのJSONデータ解析スキルを向上させてください。
ディスカッション
コメント一覧
まだ、コメントがありません