new演算子を使ったインスタンスの作成(Unity編基本)
目次
Playerクラスのインスタンスを別クラスから作成するパターン
C#の基本文法の学びに出てくるパターンですね
シーンの構成
次を参考にしましょう
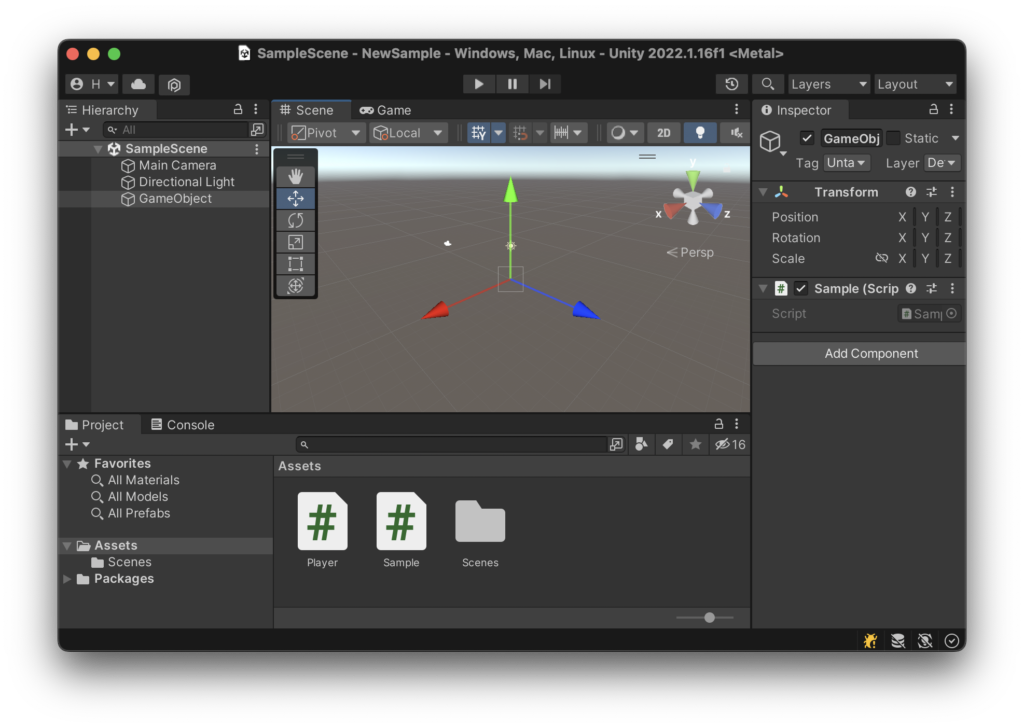
Playerクラス
インスタンスを作るためのクラスです
public class Player
{
public string name;
public int hp;
}
インスタンスを作るクラス
Playerクラスからインスタンスを作成します
using UnityEngine;
public class Sample : MonoBehaviour
{
void Start()
{
Player player1 = new Player();
player1.name = "太郎";
player1.hp = 100;
Player player2 = new Player();
player2.name = "次郎";
player2.hp = 50;
}
}
インスタンスを作成するのをさらに別クラスに移譲するパターン
new演算子を使ったインスタンスの作成を別のクラスに任せます
シーンの構成
次を参考にしましょう
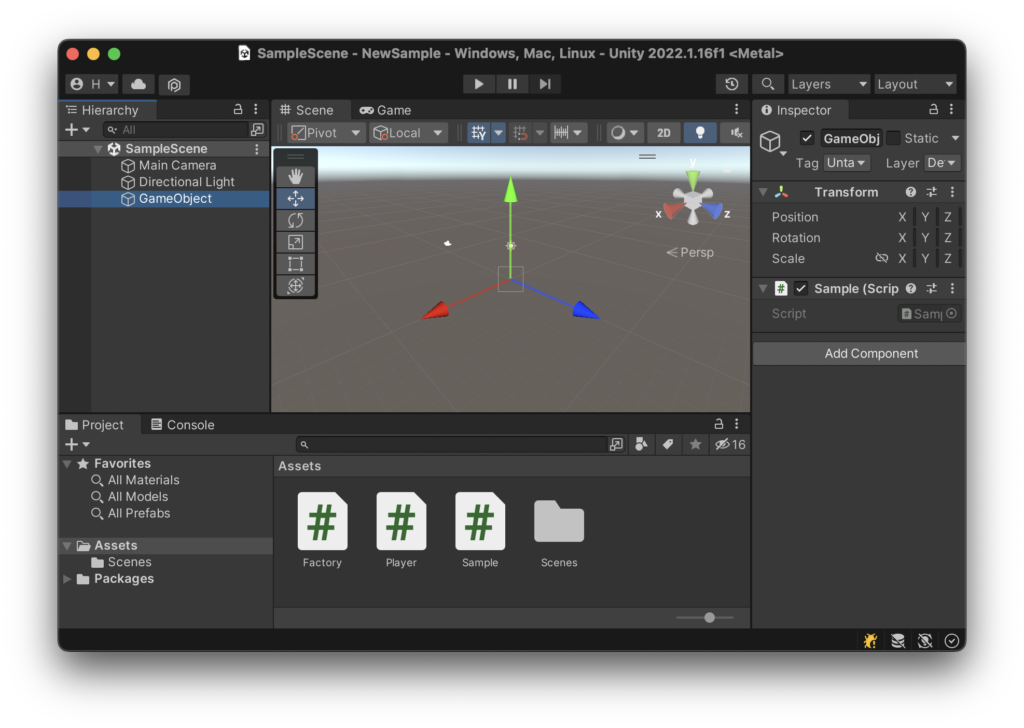
Playerクラス
インスタンスを作るためのクラスです
public class Player
{
public string name;
public int hp;
}
インスタンスを作るクラス
CreatePlayerメソッドが呼び出されると、Playerクラスからインスタンスを作成し、戻します
public static class Factory
{
public static Player CreatePlayer()
{
return new Player();
}
}
インスタンスを取得するクラス
Playerクラスからのインスタンスは別クラスに任せ、作成されたインスタンスをもらいます
using UnityEngine;
public class Sample : MonoBehaviour
{
void Start()
{
Player player1 = Factory.CreatePlayer();
player1.name = "太郎";
player1.hp = 100;
Player player2 = Factory.CreatePlayer();
player2.name = "次郎";
player2.hp = 50;
}
}
インスタンスの作成を作成するクラス自身で行うパターン
new演算子を使ったインスタンスの作成をそのクラスに任せます
シーンの構成
次を参考にしましょう
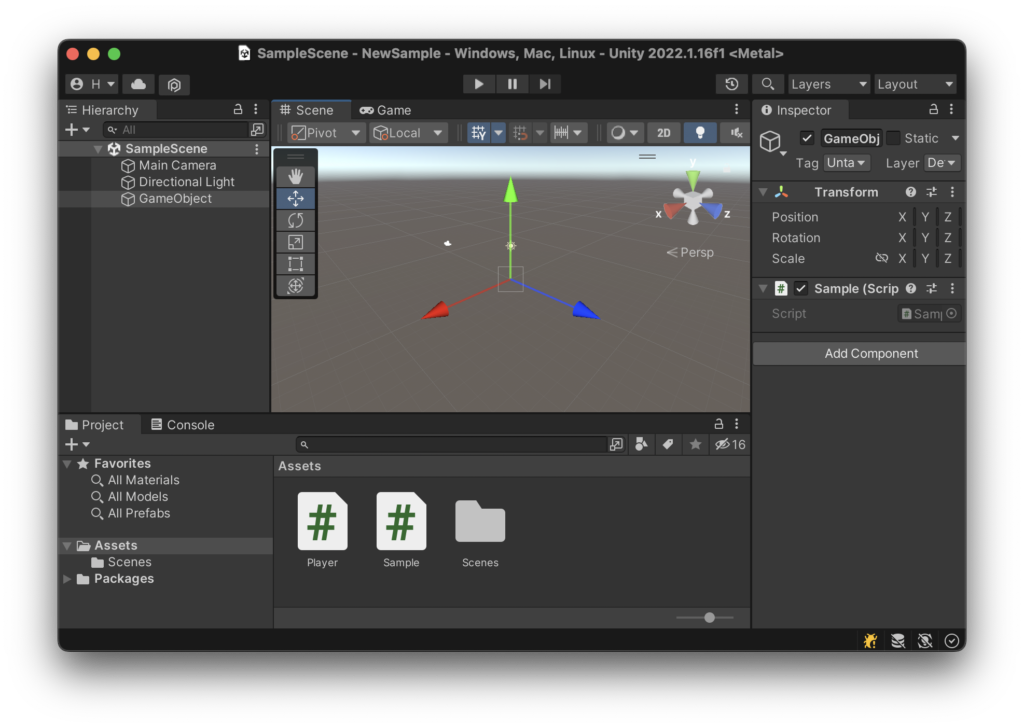
Playerクラス
インスタンスを作る元のクラスです
Playerクラスのインスタンス作成も自身で行います
public class Player
{
public string name;
public int hp;
public static Player Create()
{
return new Player();
}
}
インスタンスを取得するクラス
using UnityEngine;
public class Sample : MonoBehaviour
{
void Start()
{
Player player1 = Player.Create();
player1.name = "太郎";
player1.hp = 100;
Player player2 = Player.Create();
player2.name = "次郎";
player2.hp = 50;
}
}
テスト結果を確認するためのブレークポイント
Macでのブレークポイント設定ですが、基本Windowsでも同じです
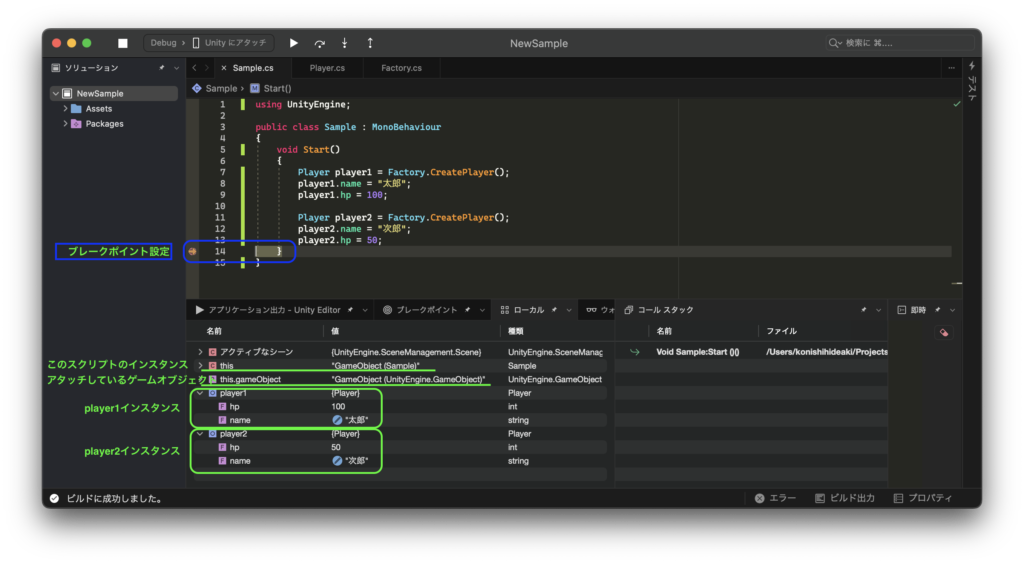
ディスカッション
コメント一覧
まだ、コメントがありません