Dictionaryの学習
学習のため、スケジュール管理の部分を作ってみましょう
目次
スケジュールのキーに日付を使ってみる
DictionaryのKey(キー)にDateTimeクラスを使って実現します
コード
// スケジュールリストを表すDictionaryを作成
var scheduleList = new Dictionary<DateTime, string>();
// スケジュールリストに要素を追加
scheduleList.Add(new DateTime(2023, 2, 1), "チームとのミーティング");
scheduleList.Add(new DateTime(2023, 2, 3), "顧客へのプレゼンテーション");
scheduleList.Add(new DateTime(2023, 2, 5), "チームビルディングイベント");
// スケジュールリストの内容を表示
foreach (var item in scheduleList)
{
Console.WriteLine($"On {item.Key.ToString("MM/dd/yyyy")}: {item.Value}");
}
結果の表示
On 02/01/2023: チームとのミーティング
On 02/03/2023: 顧客へのプレゼンテーション
On 02/05/2023: チームビルディングイベント
スケジュールをファイルに保存・読み出ししてみる
Jsonフォーマットで簡易的に保存します
コード
名前空間の追加が必要です
using System.Text.Encodings.Web;
using System.Text.Json;
using System.Text.Unicode;
// Encode設定
var op = new JsonSerializerOptions
{
// 日本語を扱う
Encoder = JavaScriptEncoder.Create(UnicodeRanges.All),
// 改行付き表示にする
WriteIndented = true
};
//json(テキスト)に変換する
string json = JsonSerializer.Serialize(scheduleList, op);
// jsonテキストをファイルに保存
File.WriteAllText("ScheduleList.json", json);
// ファイルから読み出す
string loadJsonScheduleList = File.ReadAllText("ScheduleList.json");
// オブジェクトに戻す
var loadScheduleList = JsonSerializer.Deserialize<Dictionary<DateTime, string>>(loadJsonScheduleList);
Console.ReadLine();
結果の表示
On 02/01/2023: チームとのミーティング
On 02/03/2023: 顧客へのプレゼンテーション
On 02/05/2023: チームビルディングイベント
ScheduleListファイルの内容
{
"2023-02-01T00:00:00": "チームとのミーティング",
"2023-02-03T00:00:00": "顧客へのプレゼンテーション",
"2023-02-05T00:00:00": "チームビルディングイベント"
}
ファイルの保存先
VisualStudioのソリューションエクスプローラー
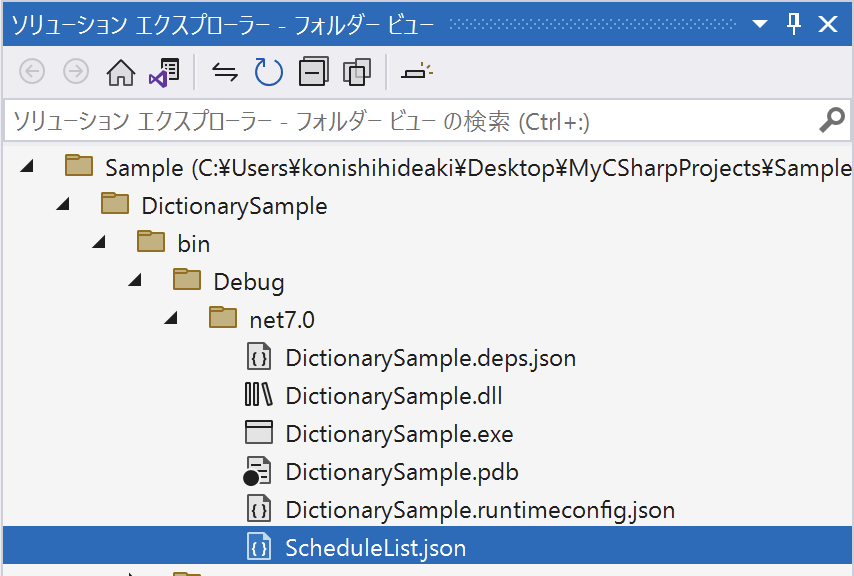
Windowsのエクスプローラー
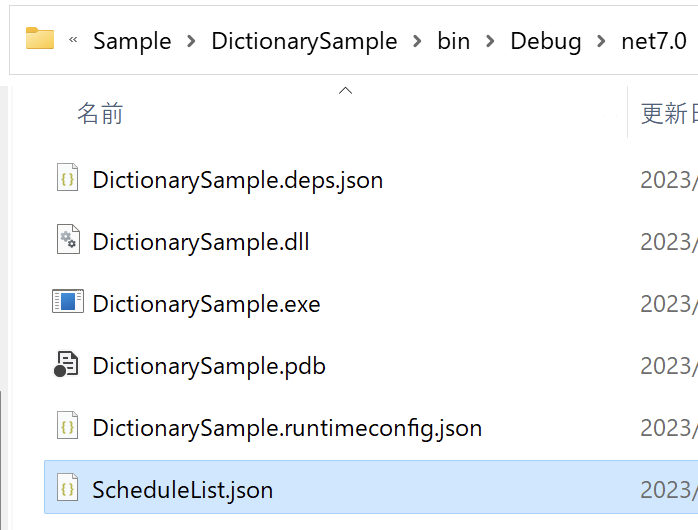
Macのフォルダ
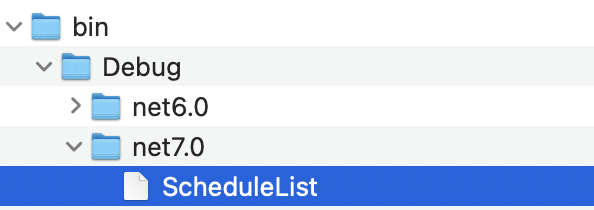
スケジュールの詳細を細かく設定できるようにする
DictionaryのValue(値)に自作のスケジュールクラスを使って実現します
その日のスケジュールについての詳細が記録できるようにしています
サンプルでは、次の属性を備えています
もちろん増やすこともできますよね
- タイトル
- 場所
- メモ
using System.Text.Encodings.Web;
using System.Text.Json;
using System.Text.Unicode;
// スケジュールリストを表すDictionaryを作成
var scheduleList = new Dictionary<DateTime, Schedule>();
// スケジュールリストに要素を追加
scheduleList.Add(new DateTime(2023, 2, 1), new Schedule("チームとのミーティング", "第1会議室", "資料1が必要"));
scheduleList.Add(new DateTime(2023, 2, 3), new Schedule("顧客へのプレゼンテーション", "第2会議室", "資料2が必要"));
scheduleList.Add(new DateTime(2023, 2, 5), new Schedule("チームビルディングイベント", "第3会議室", "資料3が必要"));
// スケジュールリストの内容を表示
foreach (var item in scheduleList)
{
Console.WriteLine($"On {item.Key.ToString("MM/dd/yyyy")}: {item.Value.Title}");
}
// Encode設定
var op = new JsonSerializerOptions
{
// 日本語を扱う
Encoder = JavaScriptEncoder.Create(UnicodeRanges.All),
// 改行付き表示にする
WriteIndented = true
};
//json(テキスト)に変換する
string json = JsonSerializer.Serialize(scheduleList, op);
// jsonテキストをファイルに保存
File.WriteAllText("ScheduleList.json", json);
// ファイルから読み出す
string loadJsonScheduleList = File.ReadAllText("ScheduleList.json");
// オブジェクトに戻す
var loadScheduleList = JsonSerializer.Deserialize<Dictionary<DateTime, Schedule>>(loadJsonScheduleList);
Console.ReadLine();
internal class Schedule
{
public Schedule(string title, string place, string memo)
{
Title = title;
Place = place;
Memo = memo;
}
public string Title { get; set; }
public string Place { get; set; }
public string Memo { get; set; }
}
結果の表示
On 02/01/2023: チームとのミーティング
On 02/03/2023: 顧客へのプレゼンテーション
On 02/05/2023: チームビルディングイベント
ディスカッション
コメント一覧
まだ、コメントがありません